Google Pay™ for Web Implementation
Learn how to implement Google Pay for Web.
Preczn's Google Pay client is a client-side (browser) script which reduces the complexity of integrating to Google Pay for Web for transaction processing with Preczn.
You can add the Google Pay button to your web interface and then initialize it's functionality using a secure Preczn-hosted JavaScript client. Once an Google Pay payment method is obtained the Preczn client generates a secure single-use merchant-specific token, which you can then safely transmit from your client-side web application to your server-side for use processing a transaction.
📱 Implementing Google Pay
For a quick implementation crash course on the Preczn Google Pay client, see the following recipe:
1. Add the Google Pay button to your page
Include the Google Pay JavaScript SDK and define the button element
📖 Reference: Follow the Google Pay Developer Documentation guide for User experience best practices.
⚠️ There are several options for producing a Google Pay button on your web interface. Preczn only supports the google-pay-button
web component for which all resources are included by the Preczn client.
Add the <google-pay-button>
element on your page where you would like it displayed:
<google-pay-button button-color="default" button-type="buy"></google-pay-button>
Be sure to create exactly one
google-pay-button
elementThe Preczn Google Pay client will automatically discover the
<google-pay-button>
element on your page (usinggetElementsByTagName('google-pay-button')
) and will render errors if zero or more than one<google-pay-button>
elements are discovered.
Customize the Google Pay button
🎨 Customize the look and feel of the Google Pay button with CSS and <google-pay-button>
element properties! See Google Pay: Customize your button for details and convenient tools for customizing the Google Pay button.
Ensure that you select the "Web components" library when using this tool to help customize your Google Pay button:
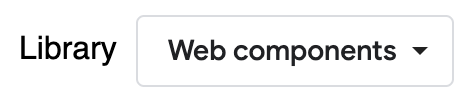
❗Your website must comply with the Google Pay acceptable policy. For more information, see Google's Acceptable Use Policy for Google Pay API.
2. Include the Preczn client on your page
Add the secure Preczn JavaScript client reference to the <head>
of your page:
<script fetchPriority="high" src="https://api.preczn.com/v1/clients/preczn.min.js?resources=googlepay&merchantId=mid_2zyd88xrnr90xskmjmqpd0x1vj&publicApiKey=2482re32338cd9z5048v7bhmb5"></script>
The following query parameters appended the script src
URL are required:
Parameter | Description |
---|---|
merchantId | The Preczn merchant ID for which you want to display the Google Pay button. |
publicApiKey | Your platform public API key for authentication. |
resources | A comma-delimited list of the client-side resources which you choose to utilize from the Preczn client. For Google Pay, include googlepay in this value. |
Public Key Authentication
Ensure that you're using a public API key with PaymentFields, and not exposing and potentially compromising a sensitive private API key!
2.1. (Optional) Confirm all requisite Google Pay resources have loaded and initialized
Preczn provides 2 mechanisms to ensure the Preczn client and the Google Pay SDK have completed loading and initialization:
- The
Preczn.GooglePay.ready
value.- If
Preczn?.GooglePay?.ready
istrue
you can proceed to initialize the Google Pay button with the Preczn client. - If the value is
false
orundefined
then the resources have not yet loaded.
- If
- A
Preczn.GooglePay.ready
event on thedocument
object.- When the Preczn client has completed loading requisite resources (such as the Google Pay SDK) and initialization, it will dispatch an
Event
of the typePreczn.GooglePay.ready
on thedocument
. - Add an event listener for the
Preczn.GooglePay.ready
event ondocument
to be notified when loading and initialization have completed, then initialize the Google Pay button .document.addEventListener( "Preczn.GooglePay.ready", (e) => { // Preczn and Google Pay SDKs loaded, initialize Google Pay button Preczn.GooglePay.initGooglePayButton(123, callback); }, false, );
- ℹ️ If you subscribe to the
Preczn.GooglePay.ready
event, be sure to add your event listener todocument
before including the Preczn client on your page to avoid any chance the event is dispatched before the event listener is defined.
- When the Preczn client has completed loading requisite resources (such as the Google Pay SDK) and initialization, it will dispatch an
3. Define the required JavaScript callback function
Define a JavaScript callback function to handle tokenization result or errors:
var googlepayCallback = function (result, errors) {
if (!errors) {
// Utilize resulting Preczn single-use token here
var precznToken = result.token;
} else {
console.error(`Google Pay errors:\r\n - ${errors.join("\r\n - ")}`);
}
};
Callback function parameters
If no errors occurred the errors
parameter value will be null
, otherwise errors
will contain an array of strings describing any error conditions.
Upon success Preczn creates a single-use merchant-specific token representing the Google Pay payment method and provides it in the callback result
object. The callback result
parameter object will consist of following properties:
Property | Description |
---|---|
walletType | The walletType property of the single use token which was created. Always GOOGLEPAY . |
type | The account type of the card which the token represents: CREDIT or DEBIT . |
brand | The brand of the card which the token represents: VISA , MASTERCARD , AMEX , DISCOVER , DINERS , JCB , UNIONPAY , or OTHER . |
bin | The bank identification number (BIN) of the card which the token represents. Typically the first 6 digits of the card number. |
last4 | The last 4 digits of the card number which the token represents. |
token | The Preczn token ID for the single-use, merchant-specific token representing this Google Pay payment method. |
billingContact * | Object containing details about the billing contact associated with the payment method. |
billingContact.address | Account holder's Address line 1. |
billingContact.address2 | Account holder's address line 2. |
billingContact.city | Account holder's city. |
billingContact.region | Account holder's region or state. |
billingContact.postal | Account holder's postal or zip code. |
billingContact.country | Account holder's ISO 3166-1 alpha-3 country code. |
billingContact.email | Account holder's email address. |
* If made available by Google Pay, the billingContact
data will be included in the result
object. (Preczn always sends the value FULL
to Google Pay in the BillingAddressParameters format
request field.)
Example result
object:
{
"walletType": "GOOGLEPAY",
"type": "CREDIT",
"brand": "VISA",
"bin": "432100",
"last4": "0012",
"expiration": "1234",
"merchantId": "mid_2zyd88xrnr90xskmjmqpd0x1vj",
"token": "tkn_apkwm89dr8br9zvqw4b3xgktk",
"billingContact": {
"firstName": "Bob",
"lastName": "Smith",
"address": "1600 Amphitheatre Parkway",
"address2": "Suite 1",
"city": "Mountain View",
"region": "CA",
"postal": "94042",
"country": "USA",
"email": "[email protected]"
}
}
Google Pay Preczn Tokens are Single-use Only and Merchant-specific
Note that Preczn tokens returned for Google Pay payment methods are merchant-specific and single-use only. Due to the nature of the underlying data, a multi-use token cannot be returned when the token is used for a transaction. Therefore it is advised that these tokens not be stored as card-on-file and not be used for a
verify
type transaction. Instead these tokens should be used to conduct a 'final' transaction such as asale
type transaction.
Callback errors
🛑 The following are errors which the Preczn Google Pay client may send to your callback function:
Invalid API Key
- Invalid Preczn public API key provided in
publicApiKey
query string parameter.
- Invalid Preczn public API key provided in
Invalid Merchant ID
- Invalid Preczn merchant ID provided in
merchantId
query string parameter.
- Invalid Preczn merchant ID provided in
Unauthorized Request
- HTTP 401 or 403 response received from Preczn API during attempt to validate merchant payment session or tokenize Google Pay payment data. Confirm public API key and merchant ID values and try again. Contact Preczn support if the problem persists.
Google Pay not enabled for merchant
- The merchant has had Google Pay disabled, review the merchant settings and contact Preczn support if the problem persists.
Google Pay can only be initialized on a secure (HTTPS) connection
- Google Pay will only work when the button is served via secure HTTPS connection.
No google-pay-button elements found on page
- The Preczn Google Pay client found no
google-pay-button
elements on your page. Ensure you have onegoogle-pay-button
element on your page.
- The Preczn Google Pay client found no
Too many google-pay-button elements (N) found on page
- The Preczn Google Pay client found more than one
google-pay-button
elements on your page, whereN
is the number of matching elements found. Ensure you have only onegoogle-pay-button
element on your page.
- The Preczn Google Pay client found more than one
Failed to decrypt and tokenize Google Pay payment data
- The Preczn API failed to decrypt the Google Pay payment data and create a single-use token from the result. Try again and contact Preczn support if the problem persists.
4. Initialize the Google Pay button with the Preczn client
Call the Preczn JavaScript client to initialize the Google Pay button:
<script type="text/javascript">
Preczn.GooglePay.initGooglePayButton(123, callback);
</script>
The initGooglePayButton
function takes up to 4 parameters, in this order:
Parameter | type | Description |
---|---|---|
Amount * | Number | The amount to charge for the transaction as a whole integer ($1.00 is 100 ). |
Callback Function * | function | The function which is called when a single-use token is successfully generated for a cardholders Google Pay payment method, or upon errors. |
Country Code | string enum value | ISO 3166-1 alpha-2 country code. Defaults to US . |
Currency Code | string enum value | ISO-4217 3-character currency code. Defaults to USD . |
* Denotes a required parameter.
🧪 Testing Google Pay
Preczn Test Mode configurations utilize the Google Pay test mode sandbox. See the Google Pay mock test cards page for information regarding test card data to use for testing google pay in test mode.
Using with a Content Security Policy
If you are using a Content Security Policy to secure your client-side web application, you will need to extend your policy to allow preczn.min.js
to load and connect to the Preczn API.
Please add the following directives to your content security policy:
Directive | Value |
---|---|
script-src | api.preczn.com pay.google.com |
connect-src | api.preczn.com pay.google.com google.com |
frame-src | pay.google.com |
img-src | www.gstatic.com |
💳 Process a Google Pay Transaction
In order to process a transaction using the Google Pay data you receive from this integration, send the merchant-specific single-use token and customer billing information, which you obtained from the client-side, to the Process Transaction API endpoint.
See the following recipe for an example:
Updated about 1 month ago