PaymentFields Integration
Preczn PaymentFields is a client-side (browser) script which helps reduce the PCI scope of your software by eliminating the need for your server-side software to transmit or store payment account data.
The PaymentFields script binds to existing input controls on your payment form and generates a secure single-use token, which you can then safely transmit from your client-side web application to your server-side for use processing a transaction.
1. Include the client on your page
Add the secure, hosted JavaScript reference in the <head>
of your page:
<script src="https://api.preczn.com/v1/clients/preczn.min.js?resources=paymentfields&publicApiKey=2482re32338cd9z5048v7bhmb5"></script>
Public Key Authentication
Ensure that you're using a public API key with PaymentFields, and not exposing and potentially compromising a sensitive private API key!
2. Define the required form and controls
Add the following 3 payment information input fields to your form, or add the their respective data-preczn
attributes to your existing input fields:
<input type="text" data-preczn="number" />
<input type="text" data-preczn="expiration" />
<input type="text" data-preczn="cvv" />
Notes
- PaymentFields form controls (with
data-preczn
attributes) are prohibited from havingid
orname
attributes in order to ensure that sensitive payment data is not inadvertently posted to your servers.- The
data-preczn="number"
field is expected to be 12-19 numeric characters and a valid credit card number- The
data-preczn="expiration"
field is expected to be 4 numeric characters inMMYY
format- The
data-preczn="cvv"
field is expected to be 3-4 numeric characters
3. Define the required JavaScript callback function
Define a JavaScript callback function for token results:
var tokenCallback = function (result, errors) {
if (!errors) {
// Utilize resulting Preczn single-use token here
var precznToken = result.token;
} else {
console.error(`PaymentFields errors:\r\n - ${errors.join("\r\n - ")}`);
}
};
4. Obtain a payment token
Call the getToken()
function with your token callback function:
Preczn.PaymentFields.Card.getToken(tokenCallback);
The result object received by your callback method looks like this:
{
"type": "CREDIT",
"expiration": "1234",
"brand": "VISA",
"bin": "432100",
"last4": "0012",
"token": "tkn_apkwm89dr8br9zvqw4b3xgktk",
}
[
"Missing form field: number",
"Form controls are prohibited from having ID or NAME attributes: cvv"
]
Check out the following Recipe for an example implementation:
PaymentFields Data Flow
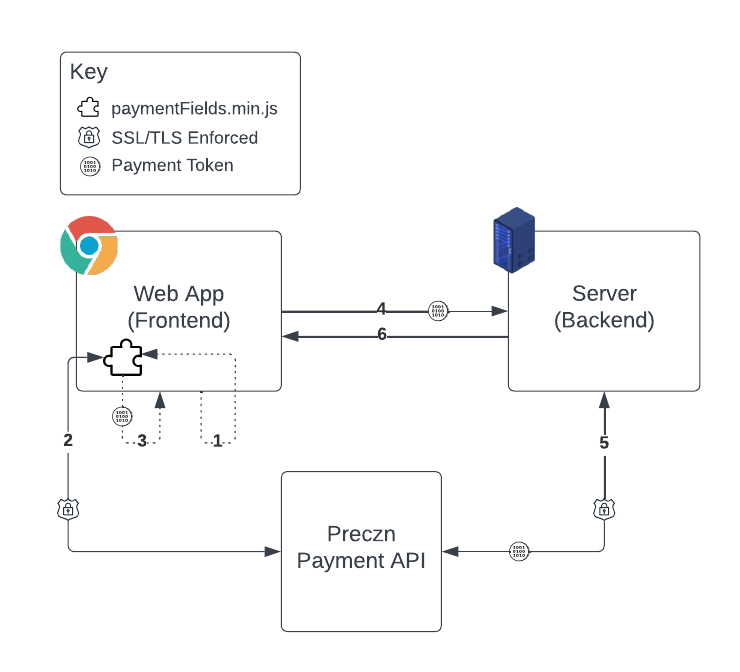
- Your frontend web application requests a payment token by calling
Preczn.PaymentFields.getToken()
. - The PaymentFields library securely calls the Preczn API to obtain a payment token.
- The PaymentFields library calls your self-defined callback function with the resulting payment token.
- Your frontend web application sends the payment token along with the rest of your order information to your backend server.
- Your backend service securely sends the transaction request with payment token to the Preczn API and receives the transaction result response.
- Your backend service returns the transaction result and order status to your frontend web application.
When properly implemented, your frontend and backend applications never touch any sensitive account data! 🚫 💳
Additional Helpful Functions!
- Validate Credit Card Number
The Preczn PaymentFields client provides a function to validate your credit card input field.
Call the Preczn.PaymentFields.Card.isValidCardNumber()
function and the Preczn client will find the input field with the data-preczn="number"
and validate that the contents are a valid credit card number.
var isValidCard = Preczn.PaymentFields.Card.isValidCardNumber();
Return Value | Description |
---|---|
null | Unable to find an input field with data-preczn="number" attribute in the document. |
true | Valid card number. |
false | Invalid card number. |
- Get Card Brand
The Preczn PaymentFields client provides a function to tell you the card brand for the value in the input field with the data-preczn="number"
attribute.
Call the Preczn.PaymentFields.Card.getBrand()
and the Preczn client will find the input field with the data-preczn="number"
and return a string representing the card brand of the current field value.
var cardBrand = Preczn.PaymentFields.Card.getBrand();
Return Value | Description |
---|---|
null | Unable to find an input field with data-preczn="number" attribute in the document or the field content length is less than 4 numeric characters. |
VISA | Visa. |
MASTERCARD | Mastercard. |
AMEX | American Express. |
DISCOVER/JCB | Discover or JCB. |
OTHER | Other unidentified card type. |
Implementation Advice
You can add real-time card brand display to your interface by calling your own function from the card number input field's
onkeyup
event property, and from that function callingPreczn.PaymentFields.Card.getBrand()
. Use the returned value to automatically display the card brand once a minimum of 4 digits have been entered into the card number field!
<script>
function displayCardBrand() {
var cardBrand = Preczn.PaymentFields.Card.getBrand();
if (cardBrand) {
// Display icon for card brand
} else {
// Hide card brand icons
}
}
</script>
<input type="text" data-preczn="number" onkeyup="displayCardBrand()" />
Use your token to process a payment
You can now use your newly acquired payment token to process a transaction from your server using the Process Transaction endpoint with a Direct API Integration!
Using with a Content Security Policy
If you are using a Content Security Policy to secure your client-side web application, you will need to extend your policy to allow paymentFields.min.js to load and connect to the Preczn API.
Please add the following directives to your content security policy:
Directive | Value |
---|---|
script-src | api.preczn.com |
connect-src | api.preczn.com |
Updated 7 months ago