PayPal Integration - Pay with PayPal
To simplify implementation of a Pay with PayPal button for your PayPal enabled merchants on Preczn, you can add the button to your web interface using a secure, Preczn-hosted JavaScript client
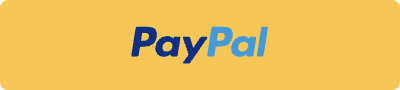
1. Include the Preczn PayPal client on your page
Add the secure, hosted JavaScript reference in the <head>
of your page:
<script src="https://api.preczn.com/v1/clients/paypal.min.js?merchantId=2zyd88xrnr90xskmjmqpd0x1vj&publicApiKey=2482re32338cd9z5048v7bhmb5"></script>
The following query parameters on the script src
URL are supported (required parameters are marked with an asterisk):
Parameter | Description |
---|---|
merchantId * | The Preczn merchant ID for which you want to display the Pay with PayPal button. |
publicApiKey * | Your platform public API key for authentication. |
intent β | PayPal-specific intent parameter, passed on to PayPal SDK.Defaults to capture when no value provided. |
commit β | PayPal-specific commit parameter, passed on to PayPal SDK.Defaults to true when no value provided. |
currency β | PayPal-specific currency parameter, passed on to PayPal SDK.Defaults to USD when no value provided. |
* Denotes a required parameter.
β Denotes a parameter passed through to the PayPal SDK. See the PayPal SDK Documentation for more information on the PayPal-specific query parameters above.
2. Define the button location
Define the location for the button on your page, we suggest containing it within a <div>
:
<div id="paypal-button-container" style="width: 200px"></div>
Note that the button's minimum width is 200px.
Its maximum width is unconstrained, and fills the available width by default.
3. Define the required JavaScript callback function
Define a JavaScript callback function to handle transaction results or errors:
var paypalCallback = function(result, errors) {
if (!errors) {
// Handle approved transaction result here
var precznTransactionId = result.id;
} else {
console.error(`Pay with PayPal errors:\r\n - ${errors.join('\r\n - ')}`);
}
}
If no errors occurred, the errors
parameter value will be null
, otherwise errors
will contain an array of strings describing any error conditions.
The callback result
parameter will be a Preczn object with the following properties:
Property | Description |
---|---|
id | The resulting Preczn transaction ID. |
amount | The amount for which the transaction was approved. |
status | The resulting transaction status, always A as this callback is only triggered upon a successfully approved transaction. |
paypalData | The PayPal result object as defined in the PayPal Orders Capture Response. |
4. Add the button
Call the Preczn JavaScript client to create the button:
<script type="text/javascript">
Preczn.PayPal.addPayPalButton("paypal-button-container", 1.23, paypalCallback);
</script>
The addPayPalButton
function takes 5 parameters, in this order:
Parameter | type | Description |
---|---|---|
Container ID * | string | The HTML DOM element ID for the element which will contain the Pay with PayPal button (we suggest containing it within a <div> element) |
Amount * | Number | The amount to charge for the transaction |
Callback Function * | function | The function to call when a Pay with PayPal transaction is successfully approved |
Items | Array of item | Array of item objects as defined in items parameter of Process Transaction. |
Currency Code | string enum value | ISO-4217 3-character currency code. Defaults to USD when omitted from function call. |
* Denotes a required parameter.
This will load all of the required scripts and stylesheets from both Preczn and PayPal into the <head>
of your page, and render the Pay with PayPal button!
The resulting button
If the Merchant for the provided merchantId
is configured with appropriate PayPal credentials, and assigned to a PayPal-enabled plan, the Pay with PayPal button will be rendered like this:
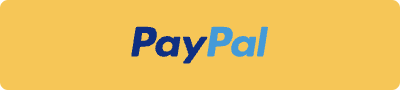
Error conditions
If any error conditions occur - such as invalid merchantId
, failed authentication, etc. - then the Preczn PayPal script will not load any PayPal dependencies or render the button on your page.
Instead, the script will write error logs to the browser console indicating that an error has occurred.
Error | Description |
---|---|
Unauthorized. | The provided publicApiKey was not valid. |
Merchant not found. | The provided merchantId was not a valid merchant ID. |
Merchant missing PayPal connection. | The merchant does not have a PayPal connection configured. |
Merchant missing PayPal Client Id. | The merchant PayPal connection is missing the requisite Client Id value. |
Merchant plan does not support PayPal. | The merchant is not assigned to a plan which supports PayPal. |
Merchant PayPal connection is disconnected. | The merchant's PayPal connection has been disconnected, check the connection on the merchant record for details. |
Using with a Content Security Policy
If you are using a Content Security Policy to secure your client-side web application, you will need to extend your policy to allow paypal.min.js to load and connect to the Preczn API, as well as to load the PayPal scripts and connect to PayPal.
Please add the following directives to your content security policy:
Directive | Values |
---|---|
script-src | api.preczn.com *.paypal.com |
connect-src | api.preczn.com *.paypal.com |
Updated over 1 year ago